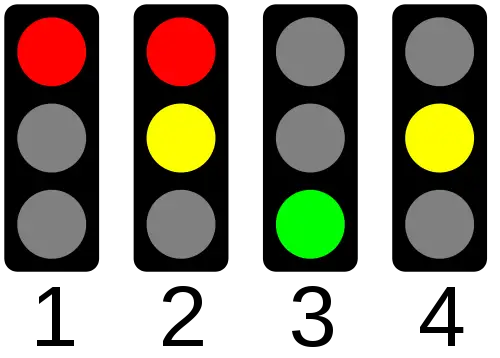
Online Link:
All my Code available on my Github
Objective
The purposes of this lab are to:
• Learn to utilize the general-purpose input/output (GPIO) pins on the Raspberry Pi to control LEDs.
• Learn to develop a minimum viable product and deliver new functionality through subsequent updates
until the requirements are met, and all of the deliverables are acceptable.
Materials
• Personal Computer
• Raspberry Pi computer
• 3x LEDs and resistors
• 4x wires
References
Index.html is copied from this CodePen
The setup of the RasberryPi and LED’s was using this Traffic Light Tutorial
The Flask Server was setup using this RaspberryPI Tutorial
Procedures
1. First let’s make sure you have ssh setup on your raspberry pi to do this you’ll need to connect it to a monitor mouse and key board. After it’s all connected open up the wifi settings and connnect to the wifi of you choice from there follow this Tutorial
2. Now that you have ssh setup ssh to your RaspberryPI and create a folder called webapp
3. We are now going to setup the python flask server using this Tutorial
4. Now that we are familiar with flask servers we are going to learn how to turn on and off the LED’s using this tutorial
5. Now comes the tricky part. Combining all three tutorials together to make one working stoplight over the python flask server. So in the python flask tutorial we learned that functions are executed based on what url is called. So what we are going to do is create paths for stop, slow, go, and cycle in the python server.
6. In the Raspberry Traffic Light Tutorial we learned how to make lights turn on and off so now we are going to add that code to the app.py file. So at the top of the file be sure to add the correct imports and then add the red, yellow and green variables defined by the pin they are connected to.
7. Now we need to create the routes for turning on each light and triggering the cycle. So add @app.route(“/stop”, methods=[‘POST’]) this will be our stop light trigger url. Under that code you can add “green.off() yellow.off() red.blink()” this will turn off the other two LED’s and make the red light blink. You can do the same for the other two methods. Now if you go in your terminal and type in “python app.py” a small webserver should start up. If you open a browser on a different computer and type in the ip address followed by “:5000/stop” the red light should start blinking
8. The cycle will be a little different from the other functions. First create app route for cycle. Once that’s created you are going to need to create a function that turns on a light waits for a few seconds and then turns it off and the next one on. And all this needs to happen continuously. To do this you can create a while loop. And under the while loop turn on a light using “green.on()” and then “sleep(number of seconds to wait)” and then turn off the light using “green.off()”. This will continue to happen forever. Once you have that code in you have a functioning Traffic light that works over wifi.
Thought Questions
- What language did you choose for implementing this project? Why?
I decided to use python and python flask for the languages because there were super easy libraries ready to go for turning on and off the LED’s and the flask server was easy to use for executing python functions. I looked into using an apache server but executing the python code was going to be pain so I decide to go python all the way.
- What is the purpose of the resistor in this simple circuit? What would happen if you omitted it?
My understanding of the resistor is to keep the amps at a bearable level for the LEDs. If too much amps go through the LED it will blow it up or in a less spectacular scenario burn it out.
- What are practical applications of this device? What enhancements or modifications would you make?
So this simple device would make it really easy to change the light when needed. So if an ambulance needed to get through a light you could change it over the internet and make sure they get through A.S.A.P. If I were to modify the device I would want to make a way for the light timing to change based on the number of cars at an intersection or maybe have different cycles depending on the time of day.
- Please estimate the total time you spent on this lab and report.
Lab: 4-5 hours Report: 2 hours
Certification of Work
I certify that the created solution represents my own work. I acknowledge openly that I borrowed code and gave credit where due. I also cited the resources used in this solution in the resources section.
Appendix
Appendix 1: System Sequence
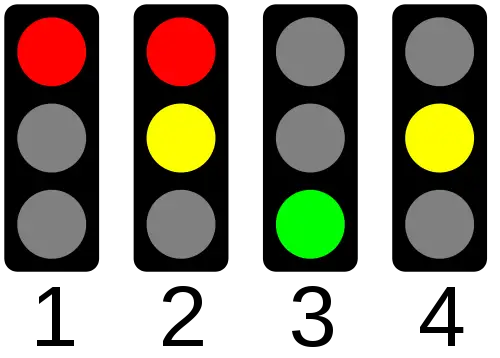
Appendix 2: Interface
Cycle Green yellow red
Appendix 3: Code
Appendix 4: Wiring
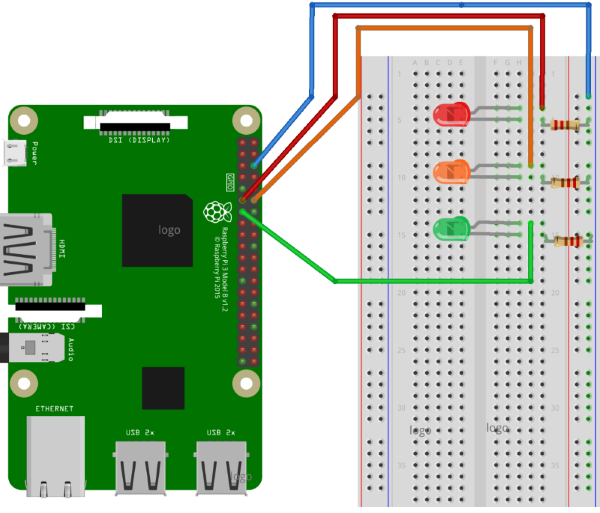